Quick Enquiry
Learn Core Java and Advanced Java at SLA Institute, the leading institute for the Core and Advanced Java Syllabus. Our syllabus covers essential topics to build a strong foundation in Java development, from basic programming concepts to advanced enterprise applications. Explore key concepts such as OOPs, multithreading, collections, JDBC, Servlets, JSP, Hibernate, and Spring Framework. Gain hands-on experience through real-world projects and practical coding exercises. SLA Institute provides expert training and career support to help you excel in Java development roles. Download our Core Java and Advanced Java Course Syllabus PDF for a detailed course structure and topics. Join our Java Course with 100% Placement Support and take the first step toward a successful career in software development. Start your journey with SLA Institute today!
Course Syllabus
Download SyllabusModule 1: Core Java Fundamentals
- Introduction to Java, Features, and JVM Architecture
- Understanding JDK, JRE, and JVM
- Data Types, Variables, and Operators
- Control Statements (Loops, Conditional Statements, Switch Case)
- Object-Oriented Programming (OOPs) Concepts – Classes, Objects, Inheritance, Polymorphism, Encapsulation, and Abstraction
- Exception Handling and Assertions (try-catch, throw, throws, finally)
- String Handling, StringBuffer, and StringBuilder Classes
- Java API Libraries and Utility Classes
Module 2: Java Collections and Multithreading
- Introduction to the Java Collections Framework
- List, Set, Map Interfaces and Their Implementations
- Comparable vs Comparator in Java
- Generics and Wrapper Classes
- Introduction to Multithreading
- Thread Life Cycle and Thread Synchronization
- Runnable Interface vs Thread Class
- Inter-Thread Communication, Deadlocks, and Concurrent Collections
Module 3: File Handling, I/O Streams, and JDBC
- Understanding Java I/O Streams (Byte and Character Streams)
- Reading and Writing Files in Java
- BufferedReader, BufferedWriter, and Scanner Class
- Serialization and Deserialization in Java
- Introduction to Database Connectivity (JDBC)
- JDBC Drivers and Connection Establishment
- CRUD Operations with MySQL/PostgreSQL
- Statement, PreparedStatement, and CallableStatement
- Connection Pooling and Transaction Management
Module 4: Java Web Development (Servlets & JSP)
- Introduction to Java EE and Web Applications
- Servlets Overview, Lifecycle, and Request-Response Model
- Handling Form Data, Request Dispatching, and URL Rewriting
- Session Management (Cookies, HttpSession, URL Rewriting)
- JSP Basics – Directives, Scripting Elements, and Expressions
- JSP Implicit Objects and Custom Tags
- Java Beans and MVC Architecture Implementation
- Creating Dynamic Web Applications with Servlets and JSP
Module 5: Advanced Java – Hibernate & Spring Framework
- Understanding ORM (Object Relational Mapping) and Hibernate Basics
- Hibernate Configuration, Mapping, and Annotations
- Hibernate Query Language (HQL) and Criteria API
- Introduction to the Spring Framework
- Spring Core Concepts – Dependency Injection and Inversion of Control (IoC)
- Spring MVC for Web Application Development
- Building RESTful Web Services using Spring Boot
- Implementing CRUD Operations in Spring Boot
Module 6: Microservices, Security, and Deployment
- Introduction to Microservices Architecture
- Creating and Managing Microservices using Spring Boot
- API Development and Integration with RESTful Services
- Implementing Security with Spring Security (OAuth, JWT)
- Introduction to Docker and Containerization for Java Applications
- CI/CD Pipeline for Java Projects (Jenkins, GitHub Actions)
- Cloud Deployment of Java Applications (AWS, Azure, Google Cloud)
Module 7: Hands-on Projects, Debugging, and Interview Preparation
- Developing a Full-Stack Java Web Application
- Implementing Authentication and Authorization in Java Projects
- Debugging, Performance Optimization, and Code Refactoring
- Best Practices for Writing Scalable and Maintainable Code
- Resume Preparation and Java Interview Questions Discussion
- Mock Interviews and Soft Skills Training
In conclusion, our Core and Advanced Java Syllabus equips learners with the essential skills to master Java programming for software development. The course covers key topics such as OOP concepts, multithreading, collections, JDBC, servlets, JSP, Hibernate, and Spring Framework, offering hands-on experience through real-world projects. With a structured curriculum, students will gain proficiency in building scalable applications, integrating databases, and developing enterprise solutions. This Core Java and Advanced Java Course Syllabus is designed to prepare learners for careers in software development, backend engineering, and full-stack Java development. Start your journey today and become an expert in Java!
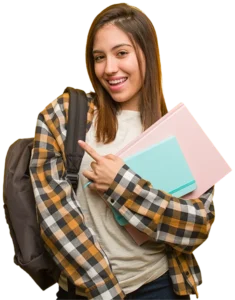